One of the more common error message in MySQL goes like this: “ERROR 1136 (21S01): Column count doesn’t match value count at row 1“.
This error typically occurs when you’re trying to insert data into a table, but the number of columns that you’re trying to insert don’t match the number of columns in the table.
In other words, you’re either trying to insert too many columns, or not enough columns.
To fix this issue, make sure you’re inserting the correct number of columns into the table.
Alternatively, you can name the columns in your INSERT
statement so that MySQL knows which columns your data needs to be inserted into.
The error can also occur if you pass the wrong number of columns to a ROW()
clause when using the VALUES
statement.
Example of Error
Suppose we have the following table:
+----------+----------+----------+ | column_0 | column_1 | column_2 | +----------+----------+----------+ | 1 | 2 | 3 | | 4 | 5 | 6 | +----------+----------+----------+
The following code will cause the error:
INSERT INTO t1 VALUES (7, 8, 9, 10);
Result:
ERROR 1136 (21S01): Column count doesn't match value count at row 1
In this case, I tried to insert data for four columns into a table that only has three columns.
We’ll get the same error if we try to insert too few columns:
INSERT INTO t1 VALUES (7, 8);
Result:
ERROR 1136 (21S01): Column count doesn't match value count at row 1
Solution 1
The obvious solution is to insert the correct number of rows. Therefore, we could rewrite our code as follows:
INSERT INTO t1 VALUES (7, 8, 9);
Result:
Query OK, 1 row affected (0.00 sec)
Solution 2
Another way of doing it is to explicitly name the columns for which we want to insert data. This technique can be used to insert less columns than are in the table.
Example:
INSERT INTO t1 (column_0, column_1) VALUES (7, 8);
Result:
Query OK, 1 row affected (0.00 sec)
This method may result in a different error if there are any constraints that require a value to be passed for that column (for example, if the table has a NOT NULL
constraint on that column). Therefore, you’ll need to ensure you’re complying with any constraints on the column when doing this.
DROP TABLE IF EXISTS student;
CREATE TABLE student(
bannerid VARCHAR(9) PRIMARY KEY NOT NULL ,
lastname VARCHAR(45) NOT NULL ,
firstname VARCHAR(45) NOT NULL ,
major VARCHAR(45) NOT NULL DEFAULT 'undeclared' ,
gpa DECIMAL(2) NOT NULL DEFAULT 0.00 ,
age INT NOT NULL DEFAULT 18 );
INSERT INTO student VALUES ('b00001111', 'smith', 'fred', 'computer science', 3.12, 20);
INSERT INTO student VALUES ('b00002222', 'jones', 'herb', 'computer science', 2.00, 19);
INSERT INTO student VALUES ('b00003333', 'chan', 'jackie', 'computer information systems', 3.50, 50);
INSERT INTO student VALUES ('b00004444', 'baker', 'al');
INSERT INTO student VALUES ('b00005555', 'booker', 'sue');
This results in the following error and I do not understand why. I want the last two INSERTs to use the default values.
MySQL Error 1136:Column count does not match value count at row 1
I’m trying to insert the following info on MYSQL but keep getting the above error? Even if I just try to insert one of the values it keeps erroring out.
CREATE TABLE depositor
(depositor_name char(30),
depositor_number varchar(20),
PRIMARY KEY (depositor_number),
FOREIGN KEY (depositor_name) REFERENCES depositor(depositor_name));
INSERT INTO depositor VALUES("Johnson", "A-101");
INSERT INTO depositor VALUES("Smith", "A-215");
INSERT INTO depositor VALUES("Hayes", "A-102");
INSERT INTO depositor VALUES("Hayes", "A-101");
INSERT INTO depositor VALUES("Turner", "A-305");
INSERT INTO depositor VALUES("Johnson", "A-201");
INSERT INTO depositor VALUES("Jones", "A-217");
INSERT INTO depositor VALUES("Lindsay", "A-222");
INSERT INTO depositor VALUES("Majeris", "A-333");
INSERT INTO depositor VALUES("Smith", "A-444");
SELECT * FROM DEPOSITOR
afuzzyllama
6,5385 gold badges47 silver badges64 bronze badges
asked Oct 15, 2012 at 17:05
2
Just in case it helps anybody.
I was getting this error while firing insert and updates on table X. There was nothing wrong with the insert and update queries. I found out that there was trigger inserting the row being updated/inserted to other table Y. It didn’t have equal number of columns. So it was throwing this error. Once I changed the table structure of Y, the error was resolved.
answered Nov 7, 2014 at 10:09
2
Replace double quotes by single quotes:
INSERT INTO depositor VALUES('Johnson', 'A-101');
answered Oct 15, 2012 at 17:12
TotoToto
89.6k62 gold badges89 silver badges126 bronze badges
3
The foreign key is referencing itself, which doesn’t make any sense (actually I’m surprised that MySQL allowed you to create it: I get an error when I try; perhaps your CREATE TABLE
command failed without you noticing and you are attempting to INSERT
into some previous version of the table which does not have two columns?).
Remove the foreign key constraint (and DROP
any existing table).
answered Oct 15, 2012 at 17:20
eggyaleggyal
123k18 gold badges213 silver badges238 bronze badges
1
When trying to insert a new data row into a table, you might run into this error:
Column count doesn't match value count at row 1.
That error message typically means the number of values provided in the INSERT
statement is bigger or smaller than the number of columns the table has, while at the same time, you did not specify the columns to be inserted. So MySQL doesn’t know which data to insert in which column and it throws back the error.
For example, you have this table employees
:
CREATE TABLE employees (
emp_no int(11) NOT NULL,
birth_date date NOT NULL,
first_name varchar(14) NOT NULL,
last_name varchar(16) NOT NULL,
gender enum('M','F') NOT NULL,
hire_date date NOT NULL,
email text,
PRIMARY KEY (emp_no);
And you try to insert a new data rows into that table with this INSERT
statement:
INSERT INTO employees
VALUES('400000', '1990-09-09', 'Joe', 'Smith', 'M', '2009-09-11');
As you can see, there are 7 columns in the table employees
but you are providing only 6 values in the INSERT
statement. MySQL returns the error:
Column count doesn't match value count at row 1
To fix this
1. Provided the full required data
If you omit the column names when inserting data, make sure to provide a full row of data that matches the number of columns
INSERT INTO employees
VALUES('400000', '1990-09-09', 'Joe', 'Smith', 'M', '2009-09-11', '[email protected]');
Or if the email
field is empty:
INSERT INTO employees
VALUES('800000', '1990-09-09', 'Joe', 'Smith', 'M', '2009-09-11', '');
2. Specify the columns to be inserted in case not all columns are going to have value.
INSERT INTO employees.employees (emp_no, birth_date, first_name, last_name, gender, hire_date)
VALUES('400000', '1990-09-09', 'Joe', 'Smith', 'M', '2009-09-11');
Sometimes, all the values are provided but you still see this error, you likely forgot to use the delimiter between two particular values and make it appear as one value. So double-check the delimiter and make sure you did not miss any semicolon.
Need a good GUI tool for databases? TablePlus provides a native client that allows you to access and manage Oracle, MySQL, SQL Server, PostgreSQL, and many other databases simultaneously using an intuitive and powerful graphical interface.
Download TablePlus for Mac.
Not on Mac? Download TablePlus for Windows.
On Linux? Download TablePlus for Linux
Need a quick edit on the go? Download TablePlus for iOS
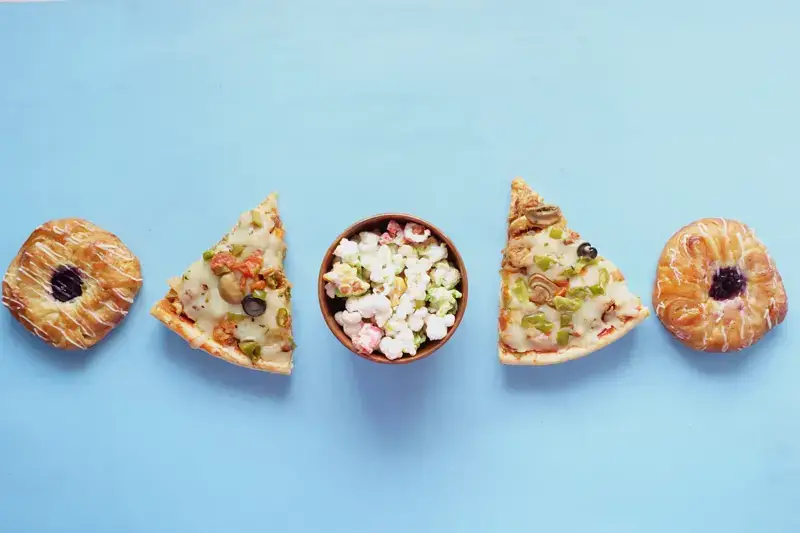
When you run an INSERT
statement, you might see MySQL responding with Column count doesn't match value count at row 1
error.
This error occurs when the number of columns that your table has and the number of values you try to insert into the table is not equal.
For example, suppose you have a pets
table with 4 columns as follows:
+----+--------+---------+------+
| id | owner | species | age |
+----+--------+---------+------+
| 1 | Jessie | bird | 2 |
| 2 | Ann | duck | 3 |
| 3 | Joe | horse | 4 |
| 4 | Mark | dog | 4 |
| 5 | Ronald | cat | 1 |
+----+--------+---------+------+
When you want to insert data into the pets
table above, you need to provide values for all 4 columns, or you will trigger the column count doesn't match value count
error:
The following INSERT
statement only provides one value for the table:
INSERT INTO pets
VALUES("Jack");
But since MySQL requires 4 values for each column, the error gets triggered:
ERROR 1136 (21S01): Column count doesn't match value count at row 1
To resolve this error, you can either:
- Provide values equal to the number of columns you have in your table
- Or specify the column table you wish to insert values
Let’s learn how to do both.
First, you can specify the values for each column as in the following statement:
INSERT INTO pets
VALUES(NULL, "Jack", "duck", 2);
The above statement will work because the pets
table has four columns and four values are provided in the statement.
But if you only have values for specific columns in your table, then you can specify the column name(s) that you want to insert into.
Here’s an example of inserting value only to the species
column. Note that the column name is added inside parentheses after the table name:
INSERT INTO pets(`species`)
VALUES("panda");
You can put as many column names as you need inside the parentheses.
Here’s another example of inserting data into three columns:
INSERT INTO pets(`owner`, `species`, `age`)
VALUES("Bruce", "tiger", 5);
The id
column is omitted in the example above.
Keep in mind that the same error will happen if you have more values to insert than the table columns.
Both statements below will return the error:
-- 👇 trying to insert five values
INSERT INTO pets
VALUES(NULL, "Jack", "duck", 2, TRUE);
-- 👇 trying to insert two values, but specify three columns
INSERT INTO pets(`owner`, `species`, `age`)
VALUES("Jane", "cat");
By default, MySQL will perform an INSERT
statement expecting each column to have a new value.
When you specify the column names that you want to insert new values into, MySQL will follow your instructions and remove the default restrictions of inserting to all columns.
And those are the two ways you can fix the Column count doesn't match value count at row 1
error in MySQL database server.
I’ve also written several articles on fixing other MySQL errors. I’m leaving the links here in case you need help in the future:
- Fix MySQL Failed to open file error 2
- Fix MySQL skip grant tables error
- Solve MySQL unknown column in field list error
And that’s it for column count doesn’t match value count error.
Thanks for reading! 👍