You’re trying to access a string
as if it were an array, with a key that’s a string
. string
will not understand that. In code we can see the problem:
"hello"["hello"];
// PHP Warning: Illegal string offset 'hello' in php shell code on line 1
"hello"[0];
// No errors.
array("hello" => "val")["hello"];
// No errors. This is *probably* what you wanted.
In depth
Let’s see that error:
Warning: Illegal string offset ‘port’ in …
What does it say? It says we’re trying to use the string 'port'
as an offset for a string. Like this:
$a_string = "string";
// This is ok:
echo $a_string[0]; // s
echo $a_string[1]; // t
echo $a_string[2]; // r
// ...
// !! Not good:
echo $a_string['port'];
// !! Warning: Illegal string offset 'port' in ...
What causes this?
For some reason you expected an array
, but you have a string
. Just a mix-up. Maybe your variable was changed, maybe it never was an array
, it’s really not important.
What can be done?
If we know we should have an array
, we should do some basic debugging to determine why we don’t have an array
. If we don’t know if we’ll have an array
or string
, things become a bit trickier.
What we can do is all sorts of checking to ensure we don’t have notices, warnings or errors with things like is_array
and isset
or array_key_exists
:
$a_string = "string";
$an_array = array('port' => 'the_port');
if (is_array($a_string) && isset($a_string['port'])) {
// No problem, we'll never get here.
echo $a_string['port'];
}
if (is_array($an_array) && isset($an_array['port'])) {
// Ok!
echo $an_array['port']; // the_port
}
if (is_array($an_array) && isset($an_array['unset_key'])) {
// No problem again, we won't enter.
echo $an_array['unset_key'];
}
// Similar, but with array_key_exists
if (is_array($an_array) && array_key_exists('port', $an_array)) {
// Ok!
echo $an_array['port']; // the_port
}
There are some subtle differences between isset
and array_key_exists
. For example, if the value of $array['key']
is null
, isset
returns false
. array_key_exists
will just check that, well, the key exists.
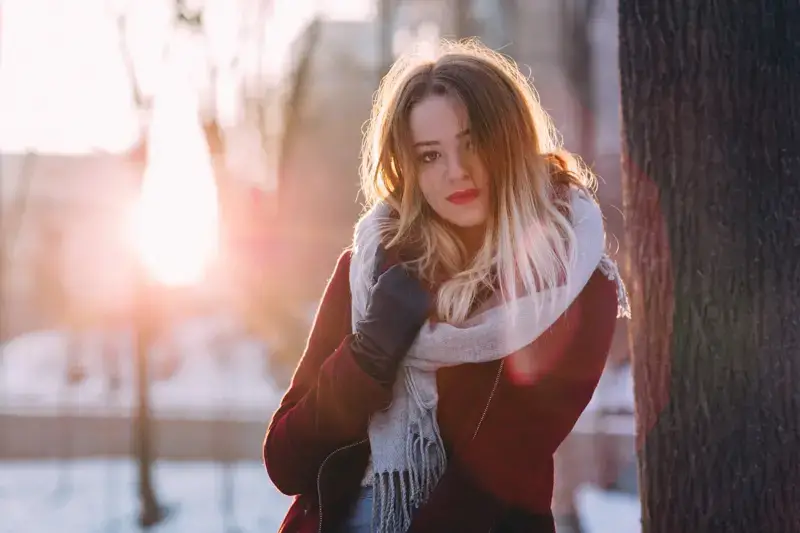
The PHP warning: Illegal string offset happens when you try to access a string
type data as if it’s an array
.
In PHP, you can access a character from a string
using the bracket symbol as follows:
$myString = "Hello!";
// 👇 access string character like an array
echo $myString[0]; // H
echo $myString[1]; // e
echo $myString[4]; // o
When you try to access $myString
above using an index that’s not available, the PHP warning: Illegal string offset occurs.
Here’s an example:
$myString = "Hello!";
// 👇 these cause illegal offset
echo $myString["state"]; // PHP Warning: Illegal string offset 'state' in..
echo $myString["a"]; // PHP Warning: Illegal string offset 'a' in ..
To resolve the PHP Illegal string offset warning, you need to make sure that you are accessing an array instead of a string.
To check if you’re actually accessing an array, you can call the is_array()
function before accessing the variable as follows:
$myArray = [
"state" => true,
"port" => 1331,
];
// 👇 check where myArray is really an array
if (is_array($myArray)) {
echo $myArray["port"];
echo $myArray["L"];
}
This warning will also happen when you have a multi-dimensional PHP array where one of the arrays is a string
type.
Consider the following example:
$rows = [
["name" => "John"],
"",
["name" => "Dave"]
];
foreach ($rows as $row) {
echo $row["name"]. "\n";
}
The $rows
array has a string at index one as highlighted above.
When you loop over the array, PHP will produce the illegal string offset as shown below:
John
PHP Warning: Illegal string offset 'name' in ...
Dave
To avoid the warning, you can check if the $row
type is an array before accessing it inside the foreach
function:
foreach ($rows as $row) {
if(is_array($row)){
echo $row["name"]. "\n";
}
}
When the $row
is not an array, PHP will skip that row and move to the next one.
The output will be clean as shown below:
When you see this warning in your code, the first step to debug it is to use var_dump()
and see the output of the variable:
From the dump result, you can inspect and see if you have an inconsistent data type like in the $rows
below:
array(3) {
[0]=>
array(1) {
["name"]=>
string(4) "John"
}
[1]=>
string(0) ""
[2]=>
array(1) {
["name"]=>
string(4) "Dave"
}
}
For the $rows
variable, the array at index [1]
is a string instead of an array.
Writing an if
statement and calling the is_array()
function will be enough to handle it.
And that’s how you solve the PHP warning: Illegal string offset. Good luck! 👍
Warning: illegal string offset in PHP seven is a common error that occurs when you try to use a string like a PHP array. This error can arise from typographical errors or a misunderstanding of the limitations of array operations on a string and that’s what this article will clear up for you.
As you read this article, we’ll show you code examples with code comments that explain what’s wrong with the code and why it won’t work. Now, grab your computer and launch your code editor because you’re about to learn how to fix illegal string offset in PHP.
Contents
- Why PHP Reports an Illegal String Offset in Your Code
- – You Accessed a String Like an Array
- – You Initialize a Variable as a String
- – Your Multidimensional Array Has a String Element
- – Your Variable Contains Json Encoded Data
- How To Stop Illegal String Offsets in PHP
- – Use Conditional Statements
- – Change the Variable to an Array
- – Use “is_array()” When You Loop Multidimensional Arrays
- – Decode the Json String Before You Access It
- Conclusion
Why PHP Reports an Illegal String Offset in Your Code
PHP Reports an illegal string offset in your code because of the following:
- You accessed a string like an array
- You initialize a variable as a string
- Your multidimensional array has a string element
- Your variable contains JSON encoded data
– You Accessed a String Like an Array
You can do some array-like operations on a string in PHP, but you’ll run into errors if you try to use the string like a full PHP array. In the following code, you’re telling PHP to return the element at the “string” offset. This is illegal because that offset does not exist in the string, and PHP seven will raise a warning. A similar approach will cause the illegal string offset CodeIgniter warning.
$sample_variable = “This is a string!”;
// Access it like an array? It won’t work!
echo $sample_variable[‘string’];
?>
– You Initialize a Variable as a String
Array initialization as a string will lead to a PHP seven warning when you perform other array operations on the string. That’s because it’s not allowed and it arises as a result of forgetfulness or typographical errors. In the following code, we initialized the “array” as a string, as a result, it’ll lead to the illegal string offset PHP 7 warning.
// This is a string!, or do you want an
// array?
$sample_variable = ”;
// A mistake to assign an index to the string
// leads to an error!
$sample_variable[“why?”] = “take that!”;
// The code will not reach this point!
print_r($sample_variable);
?>
The following is another example that has an array that does not exist in the function. That’s because the variable that should hold the array is a string.
function show_internal_array_elements() {
// This is a string and not an array.
$array = ”;
$array[‘first_element’] = ‘Hello’;
$array[‘second_element’] = ‘World’;
return print_r($array);
}
show_internal_array_elements();
?>
– Your Multidimensional Array Has a String Element
Multidimensional arrays that contain string as an array element will lead to the warning: illegal string offset php foreach warning. This happens because when you loop over this array, you’ll check the array keys before you proceed.
But a string in-between these arrays will not have a “key” and the following is an example.
// Sample names in a multidimensional array
$sample_names = [
[“scientist” => “Faraday”],
“”,
[“scientist” => “Rutherford”]
];
// There is a string in the “$sample_names”
// multidimensional array. So, the result
// in a PHP illegal string offset.
foreach ($sample_names as $values) {
echo $values[“scientist”]. “\n”;
}
?>
– Your Variable Contains Json Encoded Data
You can’t treat JSON encoded data like an array and if you do, you’ll get a warning about an illegal offset in PHP seven. You’ll run into this error when working with Laravel and you attempt to send an object to the server. In the following code, we used an array operation on JSON encoded data, and the result is an illegal offset warning.
// An associative array
$student_scores = array(“Maguire”=>65, “Kane”=>80, “Bellingham”=>78, “Foden”=>90);
// JSON encode the array
$encode_student_scores = json_encode($student_scores, true);
// This will not work! You’ll get an illegal offset
// warning.
echo $encode_student_scores[‘Maguire’];
?>
How To Stop Illegal String Offsets in PHP
You can stop illegal string offset in your PHP code using any of the following methods:
- Use conditional statements
- Change the variable to an array
- Use “is_array()” when you loop multidimensional arrays
- Decode the JSON string before you access it
– Use Conditional Statements
Conditional statements will not get rid of the illegal offset warning in your code, but they’ll ensure PHP does not show it at all. In the following, we revisit our first example and we show how a conditional statement can show custom error messages. We use the PHP “is_array()” function to check if the variable is an array before doing anything.
$sample_variable = “This is a string!”;
// Use a combination of “is_array()” and “isset()”
// to check if “$sample_variable” is an array.
// If true, only then we print the key.
if (is_array($sample_variable) && isset($sample_variable[‘string’])) {
echo $sample_variable[‘string’];
} else {
echo “Variable is not an array or the array key does not exists.\n”;
}
// Here, we use “is_array()” and “array_key_exists”
// for the check.
if (is_array($sample_variable) && array_key_exists($sample_variable[‘string’], $sample_variable)) {
echo $sample_variable[‘string’];
} else {
echo “Second response: Variable is not an array or the array key does not exist”;
}
?>
– Change the Variable to an Array
You can’t treat strings like a full PHP array, but mistakes do happen in programming. The following is the correct example of the code that initialized a string and then performed an array-like operation.
The correction is to change the string to an array using “array()” or square bracket notation. This tells PHP that the variable is an array and it will stop the illegal string offset PHP array warning.
// Fix1: Convert “$sample_variable” into an
// Array
$sample_variable = [];
// You can also use the “array()” function
// itself as shown below:
// $sample_variable = array();
// Now, this will work!
$sample_variable[“why?”] = “take that!”;
// This time, you’ll get the content of the array!
print_r($sample_variable);
?>
Also, the following is a rewrite of the function that shows the same warning. This time, we ensure the variable is an array and not a string.
function show_internal_array_elements() {
// Change it to an array and everything
// will work
$array = [];
$array[‘first_element’] = ‘Hello’;
$array[‘second_element’] = ‘World’;
return print_r($array);
}
show_internal_array_elements();
?>
– Use “is_array()” When You Loop Multidimensional Arrays
You should confirm if an element of your multidimensional array is an array using the “is_array()” function in PHP. In the following, we’ve updated the code that showed the PHP illegal offset warning earlier in the article. So, when you loop through elements of the array, you can check if it’s an array before you print its value.
// Sample names in a multidimensional array
$sample_names = [
[“scientist” => “Faraday”],
“”,
[“scientist” => “Rutherford”]
];
// Now, the “foreach()” loop has the
// is_array() function that confirms if
// the array element is an array before
// printing its value.
foreach ($sample_names as $values) {
if (is_array($values)) {
echo $values[“scientist”]. “\n”;
} else {
echo “Not an array.\n”;
}
}
/* Expected output:
Faraday
Not an array.
Rutherford
*/
?>
– Decode the Json String Before You Access It
If you have a JSON encoded string, decode it before treating it like an array. If you’re working with Laravel, this will prevent the illegal string offset Laravel warning. The following is not a Laravel code but you can borrow the same idea to fix the warning in Laravel. The idea is to decode JSON encoded data using “json_decode()”.
// An associative array
$student_scores = array(“Maguire”=>65, “Kane”=>80, “Bellingham”=>78, “Foden”=>90);
// JSON encode the array
$encode_student_scores = json_encode($student_scores, true);
// Fix: Decode the json before you do
// anything else. Don’t forget to include the
// second argument, ‘true’. This will allow
// it to return an array and not an Object!
$JSON_decode_student_scores = json_decode($encode_student_scores, true);
// Now, this will work as expected!.
print_r($JSON_decode_student_scores[‘Maguire’]);
?>
Conclusion
This article shows you why PHP seven shows a warning about an illegal string offset in your code. Later, we explained techniques that you can use taking note of the following:
- When you treat a string like an array, it can lead to the PHP warning about illegal offset.
- Array-like operations on JSON encoded data will cause an illegal offset warning in PHP seven.
- Use the PHP “is_array()” function to check if a variable or an element is an array before working on it.
- Use conditional statements to show a custom message in place of the illegal string offset warning in PHP seven.
- Illegal string offset meaning depends on your code, it’s either you’re treating an array like a string or you did not confirm if your variable is an array.
Everything that we discussed shows that you should not always treat strings like a PHP array. With your newly found knowledge, you know how to avoid PHP warnings about illegal offsets in your code.
- Author
- Recent Posts
Your Go-To Resource for Learn & Build: CSS,JavaScript,HTML,PHP,C++ and MYSQL. Meet The Team
The «Illegal string offset» warning in PHP occurs when you try to access an offset of a string variable as if it were an array. In order to fix this, make sure you are using the correct variable type (e.g. an array) when trying to access the offset. If you want to access a specific character in a string, you can use the square brackets and the string index, starting from 0.
For example, this will not trigger the warning:
<?php
$string = 'abc';
echo $string[1]; // prints "b"
If you accidentally use a string variable where an array is expected, you can use the (array)
type casting to convert the string to an array.
<?php
// Define a string variable
$string = 'abc';
// Cast the string to an array
$string_array = (array) $string;
// Access the first character of the first element in the array
// The first index [0] accesses the first element of the array, which is a string "abc"
// The second index [0] accesses the first character of the string, which is "a"
echo $string_array[0][0]; // prints "a"
Or use the str_split()
function to convert a string to an array of single-character strings.
<?php
$string = 'abc';
$string_array = str_split($string);
echo $string_array[1]; // prints "b"
If you are sure that the variable is an array and the error is still occurring, you may want to check if the array key you are trying to access exists before trying to access it.
<?php
// $array is an array variable
$array = ["first" => "apple", "second" => "banana", "third" => "cherry"];
// $key is a string variable
$key = "second";
// Checking if the specified key exists in the array using the isset() function
if (isset($array[$key])) {
// If the key exists, access the corresponding element in the array
$value = $array[$key];
echo "The value of the key '$key' in the array is: $value";
} else {
// If the key does not exist, display a message
echo "The key '$key' does not exist in the array";
}
TL; DR
Вы пытаетесь получить доступ к string
, как если бы это был массив, с ключом, который string
. string
не поймет это. В коде мы видим проблему:
"hello"["hello"];
// PHP Warning: Illegal string offset 'hello' in php shell code on line 1
"hello"[0];
// No errors.
array("hello" => "val")["hello"];
// No errors. This is *probably* what you wanted.
Глубина
Посмотрим на эту ошибку:
Предупреждение: Недопустимое смещение строки ‘порт’ в…
Что он говорит? В нем говорится, что мы пытаемся использовать строку 'port'
как смещение для строки. Вот так:
$a_string = "string";
// This is ok:
echo $a_string[0]; // s
echo $a_string[1]; // t
echo $a_string[2]; // r
// ...
// !! Not good:
echo $a_string['port'];
// !! Warning: Illegal string offset 'port' in ...
Что вызывает это?
По какой-то причине вы ожидали array
, но у вас есть string
. Просто путаница. Возможно, ваша переменная была изменена, возможно, это никогда не было array
, это действительно не важно.
Что можно сделать?
Если мы знаем, что у нас должен быть array
, мы должны сделать базовую отладку, чтобы определить, почему у нас нет array
. Если мы не знаем, будет ли у нас array
или string
, все станет немного сложнее.
Что мы можем сделать — это все виды проверки, чтобы у нас не было уведомлений, предупреждений или ошибок с такими вещами, как is_array
и isset
или array_key_exists
:
$a_string = "string";
$an_array = array('port' => 'the_port');
if (is_array($a_string) && isset($a_string['port'])) {
// No problem, we'll never get here.
echo $a_string['port'];
}
if (is_array($an_array) && isset($an_array['port'])) {
// Ok!
echo $an_array['port']; // the_port
}
if (is_array($an_array) && isset($an_array['unset_key'])) {
// No problem again, we won't enter.
echo $an_array['unset_key'];
}
// Similar, but with array_key_exists
if (is_array($an_array) && array_key_exists('port', $an_array)) {
// Ok!
echo $an_array['port']; // the_port
}
Есть несколько тонких различий между isset
и array_key_exists
. Например, если значение $array['key']
равно null
, isset
возвращает false
. array_key_exists
просто проверит, что ключ существует.